Lesson 1,
Topic 1
In Progress
Procedure
03/07/2022
Visitor Counter
Components required:
- Knewton board – 1
- Arduino Nano – 1
- Arduino Nano Cable – 1
- OLED display – 1
- IR sensor block – 2
- Power Bank – 1
- USB to DC jack – 1
Circuit Diagram:
Arrange the circuit as shown in the image.
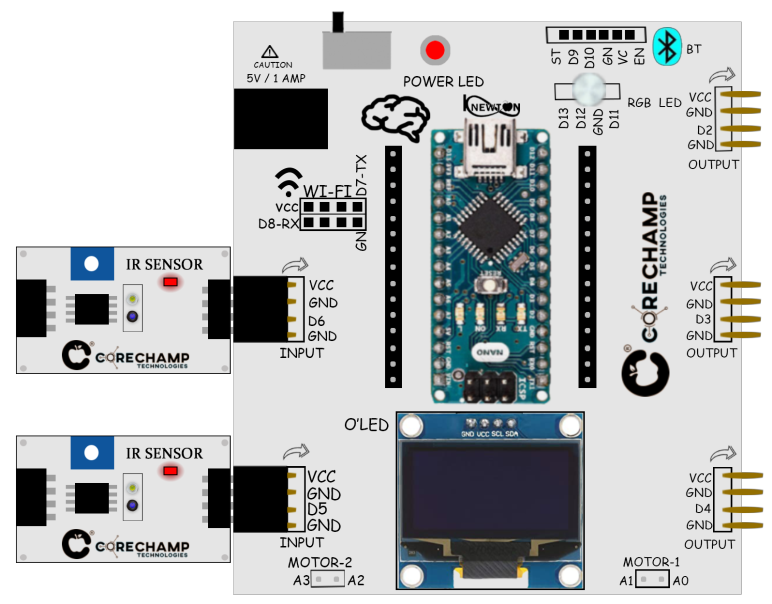
Arduino Code:
- Scan the QR to open the code. Copy the code and paste it in your Arduino IDE.
- Once you paste the code in Arduino IDE, Compile the code and upload it in the Arduino Nano.
Code:
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
//Defining the pins used
#define IR1 5
#define IR2 6
int inStatus;
int outStatus;
int countin = 0;
int countout = 0;
int in;
int out;
int now;
void setup()
{
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); //initialize with the I2C addr 0x3C (128x64)
delay(2000);
pinMode(IR1, INPUT); //Declaring IR sensor 1 at pin 5 as input device
pinMode(IR2, INPUT); //Declaring IR sensor 2 at pin 6 as input device
display.clearDisplay();
display.setTextSize(2);
display.setTextColor(WHITE);
display.setCursor(20, 20);
display.print("Visitor");
display.setCursor(20, 40);
display.print("Counter");
display.display();
delay(3000);
}
void loop()
{
inStatus = digitalRead(IR1);
outStatus = digitalRead(IR2);
if (inStatus == 0)
{
in = countin++;
}
if (outStatus == 0)
{
out = countout++;
}
now = in - out;
if (now <= 0) { display.clearDisplay(); display.setTextSize(2); display.setTextColor(WHITE); display.setCursor(0, 15); display.print("No Visitor"); display.display(); display.print(now); delay(1000); } else if (now >= 1)
{
display.clearDisplay();
display.setTextColor(WHITE);
display.setTextSize(1);
display.setCursor(15, 0);
display.print("Current Visitor");
display.setTextSize(2);
display.setCursor(50, 15);
display.print(now);
display.setTextSize(1);
display.setCursor(0, 40);
display.print("IN: ");
display.print(in);
display.setTextSize(1);
display.setCursor(70, 40);
display.print("OUT: ");
display.print(out);
display.display();
display.print(now);
delay(1000);
}
}
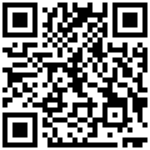
Output:
Once the code is uploaded, hover your hand above any of the IR sensors and you’ll see that the IN and OUT count displayed will change accordingly. Also the OLED will display the Total count of people present inside the room.